Advanced Web Application Security Best Practices: A Comprehensive Defense Strategy
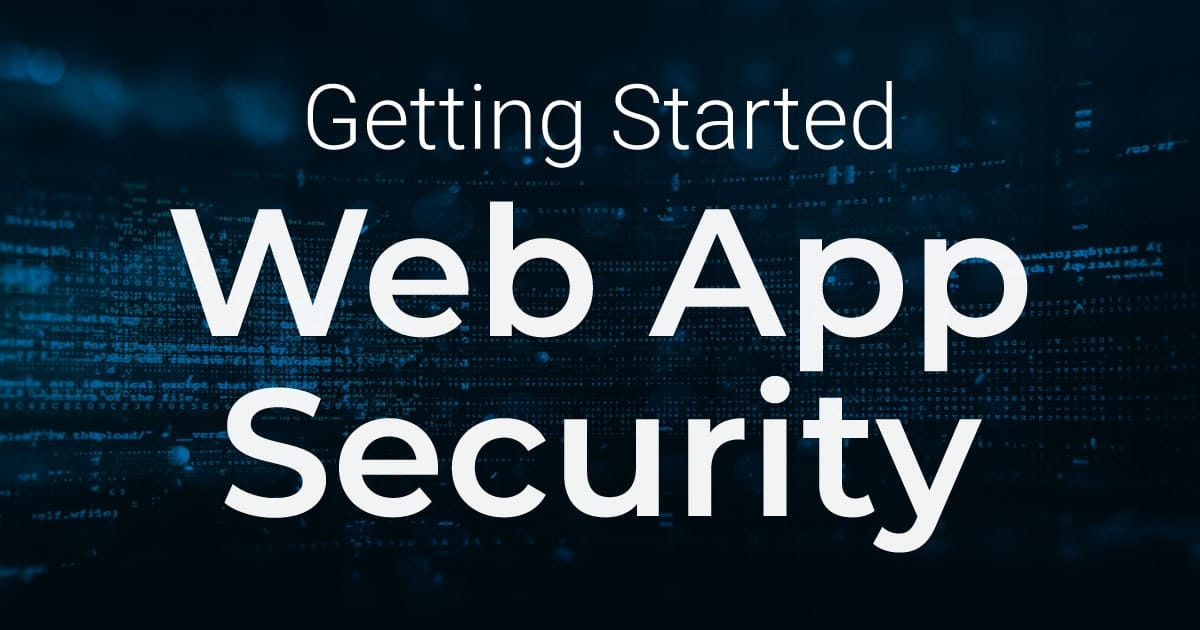
In today's interconnected digital landscape, web applications have become the primary interface between organizations and their users. As these applications handle increasingly sensitive operations and data, they've also become prime targets for sophisticated threat actors. This article provides a comprehensive examination of advanced web application security best practices, focusing on technical implementation details, architectural considerations, and emerging defense strategies that security professionals need to master in 2025.
The Evolving Web Application Threat Landscape
The threat landscape for web applications continues to evolve at a rapid pace, with attackers constantly developing new techniques to exploit vulnerabilities:
Emerging Attack Vectors
Recent threat intelligence reveals several critical trends:
- Client-side supply chain attacks targeting third-party JavaScript libraries
- API-focused exploitation leveraging insecure endpoints and authorization flaws
- Advanced injection techniques that bypass traditional WAF protections
- Server-side request forgery (SSRF) attacks targeting cloud metadata services
- WebSocket vulnerabilities in real-time applications
Understanding these evolving threats is essential for implementing effective security controls. As noted in PwnVector's research on vulnerability hunting, attackers increasingly focus on the supply chain and third-party components that traditional security measures may not adequately protect.
The Impact of Modern Development Practices
Current development methodologies significantly impact application security:
- Microservices architectures expand the attack surface through service-to-service communication
- DevOps CI/CD pipelines accelerate deployment of both features and potential vulnerabilities
- Serverless computing introduces new security considerations around function permissions
- WebAssembly (WASM) creates new client-side attack surfaces
- Progressive Web Apps (PWAs) blur the line between web and native application security
Organizations must adapt their security practices to address these architectural shifts while maintaining development velocity.
Secure Application Architecture and Design
Security must begin at the architectural level, with intentional design decisions that build defense in depth:
Zero Trust Application Architecture
Implementing zero trust principles within web applications requires several key components:
- Continuous authentication and authorization at both the request and session levels
- Fine-grained access control based on user context, behavior, and request characteristics
- Micro-segmentation between application components with explicit trust boundaries
- Complete mediation ensuring every request undergoes appropriate security checks
- Minimal privilege enforcement at every layer of the application stack
This approach dramatically reduces the impact of perimeter breaches by limiting lateral movement within the application.
Secure API Design Patterns
Modern web applications rely heavily on APIs, requiring specific security considerations:
// Example of secure API design with proper authentication, rate limiting, and input validation
app.post('/api/v1/transactions',
authenticate(), // JWT validation middleware
rateLimit({ // Rate limiting to prevent abuse
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // limit each IP to 100 requests per windowMs
standardHeaders: true, // Return rate limit info in the `RateLimit-*` headers
legacyHeaders: false, // Disable the `X-RateLimit-*` headers
}),
validateInput(transactionSchema), // Schema validation
sanitizeInput(), // Input sanitization
async (req, res) => {
// Business logic with additional authorization check
if (!await canCreateTransaction(req.user, req.body)) {
return res.status(403).json({ error: 'Unauthorized' });
}
// Proceed with transaction creation
// ...
}
);
Key API security practices include:
- Standardized authentication using OAuth 2.0 with PKCE or JWT with appropriate signing
- Comprehensive input validation for all parameters, headers, and payload data
- Output encoding specific to the context where data will be rendered
- Rate limiting and throttling to prevent abuse and denial of service
- Detailed error handling that avoids information disclosure
These controls should be implemented consistently across all API endpoints. As discussed in PwnVector's API pentesting guide, API security requires specialized testing approaches to identify vulnerabilities that might be missed by traditional scanners.
Defense in Depth Implementation
Effective web application security requires multiple layers of protection:
- Edge security: CDN-level protections, bot management, and DDoS mitigation
- Network security: Proper segmentation, TLS enforcement, and API gateways
- Application security: Input validation, output encoding, and business logic controls
- Data security: Encryption, access controls, and sensitive data handling
- Operational security: Monitoring, logging, and incident response
Each layer should operate independently while working together to provide comprehensive protection. A vulnerability in one layer shouldn't compromise the entire security model.
Critical Security Controls for Web Applications
While comprehensive security requires many controls, several are particularly critical for web applications:
Advanced Authentication Mechanisms
Modern authentication goes beyond simple username/password combinations:
- Passwordless authentication using FIDO2/WebAuthn standards
- Risk-based authentication adjusting requirements based on contextual risk factors
- Continuous authentication using behavioral biometrics and passive signals
- Multi-factor authentication (MFA) with phishing-resistant methods
// Example of WebAuthn registration in JavaScript
const publicKeyCredentialCreationOptions = {
challenge: new Uint8Array([/* challenge */]),
rp: {
name: "Example Application",
id: "example.com",
},
user: {
id: new Uint8Array([/* user ID */]),
name: "[email protected]",
displayName: "John Doe",
},
pubKeyCredParams: [
{ type: "public-key", alg: -7 }, // ES256
{ type: "public-key", alg: -257 }, // RS256
],
authenticatorSelection: {
authenticatorAttachment: "platform",
userVerification: "required",
requireResidentKey: true,
},
timeout: 60000,
attestation: "direct",
};
navigator.credentials.create({
publicKey: publicKeyCredentialCreationOptions
}).then((credential) => {
// Send credential to server for verification
}).catch((err) => {
console.error(err);
});
Implementing these advanced authentication mechanisms significantly reduces the risk of credential-based attacks like phishing and password spraying.
Content Security Policy Implementation
Content Security Policy (CSP) provides a powerful defense against XSS and other injection attacks:
Content-Security-Policy: default-src 'self';
script-src 'self' https://trusted-cdn.example.com;
style-src 'self' https://trusted-cdn.example.com;
img-src 'self' data: https://*.example.com;
connect-src 'self' https://api.example.com;
frame-ancestors 'none';
form-action 'self';
base-uri 'self';
object-src 'none';
upgrade-insecure-requests;
An effective CSP strategy includes:
- Strict source whitelisting for all resource types
- Nonce-based script execution to prevent inline script injection
- Strict dynamic for runtime script loading control
- Report-Uri configuration for monitoring and violation detection
- Incremental deployment starting with report-only mode
A properly configured CSP dramatically reduces the exploitability of XSS vulnerabilities by preventing unauthorized script execution.
Secure Session Management
Session management remains a critical security concern:
- Secure cookie attributes including SameSite=Strict, Secure, and HttpOnly flags
- Token-based session management with appropriate lifetime and rotation policies
- Session binding to client fingerprinting factors to detect session theft
- Absolute and idle timeout enforcement with appropriate session lifecycle management
- Anti-CSRF tokens for sensitive operations
// Example of secure cookie configuration
Set-Cookie: sessionId=abc123; Path=/; Domain=example.com; Max-Age=3600; HttpOnly; Secure; SameSite=Strict
Proper session management is essential for preventing session hijacking and fixation attacks. Organizations should implement comprehensive session controls and regularly audit their implementation.
Secure Coding Practices and Vulnerability Prevention
Preventing web vulnerabilities at the code level requires structured processes and secure coding practices:
Input Validation and Output Encoding
Comprehensive input validation and output encoding form the foundation of secure coding:
// Example of structured validation and encoding
function processUserInput(input) {
// Step 1: Define schema for validation
const schema = Joi.object({
username: Joi.string().alphanum().min(3).max(30).required(),
email: Joi.string().email().required(),
birthyear: Joi.number().integer().min(1900).max(2021),
role: Joi.string().valid('user', 'editor', 'admin')
});
// Step 2: Validate against schema
const { error, value } = schema.validate(input);
if (error) throw new ValidationError(error.details);
// Step 3: Sanitize input even after validation
const sanitized = {
username: sanitizeHtml(value.username),
email: sanitizeHtml(value.email),
birthyear: value.birthyear,
role: value.role
};
// Step 4: Context-specific output encoding
const htmlEncoded = {
username: encodeForHTML(sanitized.username),
email: encodeForHTML(sanitized.email),
birthyear: sanitized.birthyear,
role: sanitized.role
};
return htmlEncoded;
}
Effective strategies include:
- Input validation using schema-based approaches (JSON Schema, Joi, Yup)
- Parameterized queries for all database operations
- Context-specific output encoding for HTML, JavaScript, CSS, and URLs
- Safe deserialization practices for JSON, XML, and custom formats
- Type-safe coding patterns that leverage strong typing where possible
These practices address many common vulnerabilities, including the most prevalent web application flaws.
Security Testing Integration
Security testing must be integrated throughout the development lifecycle:
- Static Application Security Testing (SAST) integrated into IDEs and CI/CD pipelines
- Dynamic Application Security Testing (DAST) automated for critical application flows
- Interactive Application Security Testing (IAST) for runtime vulnerability detection
- Software Composition Analysis (SCA) for third-party component vulnerabilities
- API security testing specifically targeting API endpoints and business logic
# Example Jenkins pipeline with integrated security testing
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'npm install'
sh 'npm run build'
}
}
stage('SAST') {
steps {
sh 'npm run lint:security'
sh 'sonarqube-scanner'
}
}
stage('SCA') {
steps {
sh 'npm audit --audit-level=high'
sh 'snyk test'
}
}
stage('Unit Tests') {
steps {
sh 'npm test'
}
}
stage('Deploy to Test') {
steps {
sh 'docker-compose up -d'
}
}
stage('DAST') {
steps {
sh 'zap-cli quick-scan --self-contained --start-options "-config api.disablekey=true" http://localhost:3000'
}
}
}
post {
always {
junit 'test-results/*.xml'
publishHTML(target: [
allowMissing: false,
alwaysLinkToLastBuild: true,
keepAll: true,
reportDir: 'security-reports',
reportFiles: 'zap-report.html, snyk-report.html',
reportName: 'Security Reports'
])
}
}
}
This integrated approach ensures that security testing happens continuously rather than as a final gate. Implementing vulnerability testing as part of CI/CD dramatically reduces the time to remediation for identified issues.
Modern Cryptography Implementation
Proper cryptography remains challenging for many development teams:
- TLS 1.3 implementation with strong cipher suites and key exchange algorithms
- HTTPS Strict Transport Security (HSTS) to prevent downgrade attacks
- Perfect forward secrecy (PFS) to protect historical communications
- Secure key management with appropriate key rotation and storage
- Modern cryptographic algorithms for all encryption operations
# Example NGINX configuration for modern TLS settings
server {
listen 443 ssl http2;
server_name example.com;
ssl_certificate /path/to/cert.pem;
ssl_certificate_key /path/to/key.pem;
# Modern TLS configuration
ssl_protocols TLSv1.2 TLSv1.3;
ssl_prefer_server_ciphers on;
ssl_ciphers 'ECDHE-ECDSA-AES256-GCM-SHA384:ECDHE-RSA-AES256-GCM-SHA384:ECDHE-ECDSA-CHACHA20-POLY1305:ECDHE-RSA-CHACHA20-POLY1305:ECDHE-ECDSA-AES128-GCM-SHA256:ECDHE-RSA-AES128-GCM-SHA256';
# HSTS configuration
add_header Strict-Transport-Security "max-age=63072000; includeSubDomains; preload" always;
# Additional security headers
add_header X-Content-Type-Options "nosniff" always;
add_header X-Frame-Options "DENY" always;
add_header X-XSS-Protection "1; mode=block" always;
add_header Referrer-Policy "strict-origin-when-cross-origin" always;
# OCSP Stapling
ssl_stapling on;
ssl_stapling_verify on;
ssl_trusted_certificate /path/to/fullchain.pem;
resolver 8.8.8.8 8.8.4.4 valid=300s;
resolver_timeout 5s;
# Sessions and DH parameters
ssl_session_timeout 1d;
ssl_session_cache shared:SSL:50m;
ssl_session_tickets off;
ssl_dhparam /path/to/dhparam.pem;
# Other configuration
# ...
}
These cryptographic controls provide the foundation for data protection both in transit and at rest. Organizations should regularly audit their cryptographic implementations to ensure they meet current best practices.
Runtime Protection and Monitoring
Even with preventative controls, runtime protection and monitoring remain essential:
Web Application Firewall Implementation
Modern WAF deployments go beyond simple signature matching:
- Behavioral analysis to detect anomalous request patterns
- Anti-automation controls to prevent credential stuffing and scraping
- Virtual patching for zero-day vulnerability mitigation
- API-specific protections for GraphQL, REST, and other API architectures
- Machine learning models for adaptive threat detection
Effective WAF implementation requires careful tuning to balance protection against false positives, with regular rule updates based on emerging threats and application changes.
Runtime Application Self-Protection (RASP)
RASP technologies provide context-aware protection:
// Example RASP implementation (conceptual)
@Aspect
@Component
public class SecurityAspect {
@Around("execution(* com.example.controller.*.*(..))")
public Object securityCheck(ProceedingJoinPoint joinPoint) throws Throwable {
// Get request context
HttpServletRequest request = ((ServletRequestAttributes) RequestContextHolder.currentRequestAttributes()).getRequest();
// Check for suspicious patterns
if (containsSQLInjection(request.getParameterMap())) {
logSecurityEvent("SQL Injection attempt", request);
throw new SecurityException("Invalid request");
}
if (containsXSS(request.getParameterMap())) {
logSecurityEvent("XSS attempt", request);
throw new SecurityException("Invalid request");
}
// Runtime taint tracking
if (isSensitiveOperation(joinPoint)) {
trackTaintedData(joinPoint.getArgs());
}
// Proceed with execution
return joinPoint.proceed();
}
// Implementation details omitted
}
RASP solutions offer several advantages:
- Context-aware protection based on application behavior
- Zero-day vulnerability protection through runtime analysis
- Reduced false positives due to application knowledge
- Precise attack blocking at the exact vulnerability point
- Detailed security telemetry for threat analysis
Organizations should consider RASP as a complementary layer to WAF protection, particularly for critical applications with high-value assets.
Advanced Security Monitoring
Comprehensive security monitoring includes several components:
- Real-time security logging for all application components
- Behavioral analytics to detect account takeover and insider threats
- Anomaly detection for unusual application usage patterns
- Attack surface monitoring to identify exposed endpoints and assets
- Third-party risk monitoring for supply chain compromise
# Example Elasticsearch mapping for security-focused logging
PUT security-events-*/_mapping
{
"properties": {
"@timestamp": { "type": "date" },
"event": {
"properties": {
"action": { "type": "keyword" },
"category": { "type": "keyword" },
"outcome": { "type": "keyword" },
"severity": { "type": "keyword" },
"type": { "type": "keyword" }
}
},
"user": {
"properties": {
"id": { "type": "keyword" },
"name": { "type": "keyword" },
"roles": { "type": "keyword" },
"session_id": { "type": "keyword" }
}
},
"http": {
"properties": {
"request": {
"properties": {
"method": { "type": "keyword" },
"url": { "type": "keyword" },
"headers": { "type": "flattened" },
"body": { "type": "text" }
}
},
"response": {
"properties": {
"status_code": { "type": "integer" },
"headers": { "type": "flattened" },
"body_size": { "type": "long" }
}
}
}
},
"source": {
"properties": {
"ip": { "type": "ip" },
"geo": {
"properties": {
"country_name": { "type": "keyword" },
"region_name": { "type": "keyword" },
"city_name": { "type": "keyword" }
}
},
"user_agent": { "type": "keyword" }
}
},
"tls": {
"properties": {
"cipher": { "type": "keyword" },
"version": { "type": "keyword" },
"certificate_fingerprint": { "type": "keyword" }
}
},
"error": {
"properties": {
"message": { "type": "text" },
"stack_trace": { "type": "text" },
"type": { "type": "keyword" }
}
},
"tags": { "type": "keyword" }
}
}
Effective security monitoring requires integration with broader security operations center (SOC) capabilities, as outlined in PwnVector's SOC implementation guide.
Emerging Web Application Security Techniques
As threats evolve, new security techniques emerge to counter them:
Client-Side Security Controls
Client-side attacks have become increasingly sophisticated, requiring enhanced protections:
- Subresource Integrity (SRI) to prevent compromised JavaScript resources
- Trusted Types API to prevent DOM-based XSS vulnerabilities
- Feature Policy/Permissions Policy to control browser feature access
- Cross-Origin Opener Policy (COOP) and Cross-Origin Embedder Policy (COEP)
- First-Party Sets for enhanced cookie controls
<!-- Example of modern client-side security headers -->
<script src="https://cdn.example.com/library.js"
integrity="sha384-oqVuAfXRKap7fdgcCY5uykM6+R9GqQ8K/uxy9rx7HNQlGYl1kPzQho1wx4JwY8wC"
crossorigin="anonymous"></script>
<meta http-equiv="Cross-Origin-Opener-Policy" content="same-origin">
<meta http-equiv="Cross-Origin-Embedder-Policy" content="require-corp">
These browser-based controls provide an additional layer of defense, particularly against supply chain attacks targeting third-party scripts.
Zero Trust API Architecture
API security continues to evolve toward zero trust models:
- API gateway with continuous authentication for all requests
- Fine-grained authorization based on API scope and user context
- Request verification with cryptographic signatures
- Traffic profiling and anomaly detection for API abuse
- Automated API discovery and inventory management
Organizations should implement comprehensive API security governance, including API gateways, security testing, and continuous monitoring of API traffic.
Secure Front-End Frameworks
Modern front-end frameworks increasingly incorporate security by design:
- Automatic XSS prevention through template sanitization
- State management security preventing unauthorized data access
- Built-in CSRF protection for form submissions
- Default security headers for enhanced browser protections
- Component-level access controls for UI security
Development teams should leverage these built-in protections while understanding their limitations and implementing additional controls where needed.
Organizational Security Practices
Technical controls must be complemented by organizational practices:
Security Champion Programs
Scaling security expertise requires security champions embedded within development teams:
- Designated security advocates within each development team
- Specialized security training for champions
- Regular security workshops and knowledge sharing
- Security requirement refinement with champion input
- Vulnerability triage support from champions
This approach distributes security expertise throughout the organization while building a security-minded development culture.
Threat Modeling at Scale
Systematic threat modeling improves security architecture:
- Automated threat modeling integrated into design processes
- Reusable threat libraries for common application patterns
- Risk-based approach focusing on critical functionality
- Continuous threat model updates as applications evolve
- Threat model-driven security testing for validation
Organizations should implement lightweight threat modeling processes that scale with development velocity rather than becoming bottlenecks.
Bug Bounty and Vulnerability Disclosure
External security testing provides additional assurance:
- Structured vulnerability disclosure programs with clear scope and rules
- Bug bounty programs for critical applications
- Security researcher engagement for specialized testing
- Triage and remediation processes for reported vulnerabilities
- Security fix deployment pipelines for rapid response
These programs complement internal security testing by leveraging diverse expertise and perspectives.
Case Study: E-Commerce Platform Security Transformation
A major e-commerce platform implemented comprehensive web application security improvements:
Initial State
- Multiple critical vulnerabilities discovered during a penetration test
- Inconsistent security controls across microservices
- Heavy reliance on perimeter security with limited defense in depth
- Manual security testing creating deployment bottlenecks
- Poor visibility into runtime security events
Implementation Approach
The organization implemented a phased security transformation:
- Foundation phase:
- Standardized security controls across microservices
- Implemented automated security testing in CI/CD
- Deployed WAF with virtual patching capabilities
- Enhanced logging and monitoring infrastructure
- Maturity phase:
- Implemented zero trust architecture for internal services
- Deployed RASP for critical payment components
- Established security champion program
- Launched private bug bounty program
- Implemented automated threat modeling
- Optimization phase:
- Deployed advanced client-side protections
- Implemented machine learning for anomaly detection
- Developed custom security controls for business-specific risks
- Integrated security telemetry with fraud detection
- Established continuous security validation program
Results
The transformation delivered significant security improvements:
- 87% reduction in vulnerabilities discovered in production
- 95% decrease in time to remediate critical issues
- Enhanced ability to detect and respond to attacks in real-time
- Security embedded throughout the development lifecycle
- Improved resilience against emerging threats
This case study demonstrates how systematic implementation of security best practices can dramatically improve web application security while supporting business objectives.
Conclusion
Effective web application security requires a comprehensive approach that addresses people, processes, and technology. By implementing the best practices outlined in this article—from secure architecture and coding practices to runtime protection and organizational security measures—organizations can significantly reduce their vulnerability to web-based attacks.
The most successful security programs recognize that web application security is not a one-time project but a continuous process of improvement and adaptation. As threats evolve, security controls must evolve in response, requiring ongoing investment in security capabilities and expertise.
For organizations seeking to enhance their security posture, a risk-based approach focusing on the most critical vulnerabilities and assets provides the best return on security investment. By combining this prioritization with automation and integration into development processes, security can become an enabler rather than an impediment to business objectives.
Finally, collaboration between security teams, developers, and operations personnel is essential for building truly secure applications. By fostering a culture of shared responsibility for security, organizations can develop the resilience needed to thrive in an increasingly hostile threat environment.