Web Application Security Essentials: Key Protection Strategies
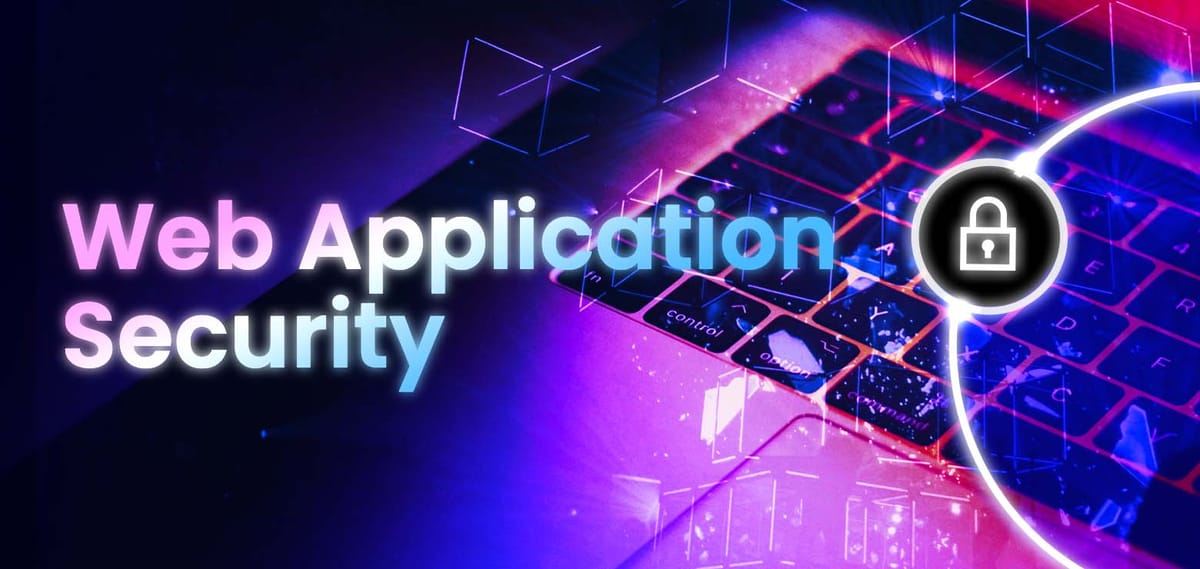
Web applications remain prime targets for attackers seeking to compromise enterprise data and systems. Despite advances in security technologies, vulnerabilities in web applications continue to present significant risks to organizations across all industries. Implementing robust security practices isn’t just a technical necessity—it’s a business imperative.
In this guide, we'll explore essential security strategies for protecting modern web applications against evolving threats. Whether you're a security professional, developer, or IT leader, these practices will help you establish effective defenses for your web applications. For more detailed approaches, see our comprehensive coverage of web application security frameworks.
Fundamental Security Strategies
Three core approaches form the foundation of effective web application security:
Defense-in-Depth Implementation
Security should never rely on a single control:
- Apply multiple protection layers (application, server, network)
- Implement both preventive and detective controls
- Ensure no single point of failure exists in security architecture
- Create overlapping security mechanisms for critical functions
This layered approach ensures that if one security control fails, others remain to protect the application. For enterprises with complex infrastructures, integrating with Active Directory security adds an important identity protection layer.
Security Integration Throughout Development
Security must be integrated across the entire application lifecycle:
- Conduct threat modeling during design phases
- Implement secure coding practices during development
- Automate security testing in build and deployment pipelines
- Deploy runtime protection for production environments
This "shift left" approach identifies vulnerabilities earlier when they're less expensive to fix. Organizations implementing DevSecOps methodologies should integrate security at every development stage.
Risk-Based Security Prioritization
Focus security efforts where they matter most:
- Prioritize controls for high-risk application components
- Address vulnerabilities based on exploitability and impact
- Implement strongest protections for sensitive data
- Balance security with business functionality requirements
This approach ensures security resources are allocated effectively to address the most significant risks first.
Essential Security Controls
The following controls represent the most critical protections for modern web applications:
Input Validation and Output Encoding
Implement thorough data validation and context-appropriate encoding:
// Node.js example using express-validator
const { body, validationResult } = require('express-validator');
app.post('/api/data', [
// Validate input
body('email').isEmail().normalizeEmail(),
body('name').isLength({ min: 2, max: 50 }).trim().escape(),
body('id').isNumeric()
], (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
// Process valid data
});
- Validate all input at the server side
- Use positive validation (allowlists) rather than negative (denylists)
- Apply context-specific output encoding
- Implement parameterized queries for database operations
These practices prevent numerous injection attacks, including the common vulnerabilities that continue to plague web applications.
Authentication and Access Control
Implement robust identity and access management:
// Express.js secure session configuration
const session = require('express-session');
app.use(session({
secret: 'strong-random-secret',
name: 'SessionId',
cookie: {
httpOnly: true,
secure: true,
sameSite: 'strict',
maxAge: 3600000
},
resave: false,
saveUninitialized: false
}));
- Deploy multi-factor authentication for sensitive functions
- Use secure password storage with modern hashing algorithms
- Implement proper session management with secure cookies
- Apply fine-grained authorization based on least privilege
- Validate authorization for every action, not just at login
Effective identity and access controls are critical defenses against account takeover and privilege escalation attacks. These measures are particularly important for preventing credential theft and abuse.
Secure API Implementation
APIs require specific security considerations:
- Authenticate and authorize every API request
- Implement rate limiting to prevent abuse
- Validate all API inputs with strict type checking
- Apply proper error handling that doesn't leak information
The rise of API-centric architectures makes API security increasingly important. Organizations should regularly test their REST APIs to identify potential vulnerabilities.
Security Headers and TLS
Implement modern security headers and proper TLS:
<!-- Key security headers -->
<meta http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self' https://trusted-cdn.example.com;">
<meta http-equiv="X-Content-Type-Options" content="nosniff">
<meta http-equiv="X-Frame-Options" content="SAMEORIGIN">
<meta http-equiv="Referrer-Policy" content="strict-origin-when-cross-origin">
- Deploy a comprehensive Content Security Policy
- Configure proper HTTPS with modern TLS (1.2+)
- Implement HTTP Strict Transport Security (HSTS)
- Use secure cookie attributes (Secure, HttpOnly, SameSite)
These transport and browser security controls provide essential protection against various attacks, including content injection and traffic interception.
Security Testing Essentials
Regular security testing is critical for identifying vulnerabilities:
Automated Security Testing
Implement automated security testing in development pipelines:
- Static Application Security Testing (SAST) to analyze source code
- Dynamic Application Security Testing (DAST) to test running applications
- Software Composition Analysis (SCA) for third-party component vulnerabilities
- Infrastructure-as-Code scanning for secure configurations
# Example GitHub Action for basic security scans
name: Security Scan
on: [push, pull_request]
jobs:
security_scan:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: SAST Scan
uses: github/codeql-action/analyze@v2
- name: Dependency Check
uses: snyk/actions/node@master
with:
args: --severity-threshold=high
Automated testing should be complemented with regular manual testing by security professionals, particularly for complex vulnerabilities that automated tools might miss.
Penetration Testing
Conduct regular penetration testing to identify vulnerabilities that automated scanning might miss:
- Focus on authentication and authorization mechanisms
- Test business logic for security flaws
- Validate input handling and output encoding
- Verify session management security
Professional penetration testing provides valuable insights into how applications behave under real attack conditions. For targeted testing, consider advanced penetration testing approaches that address specific vulnerability classes.
Runtime Protection
Beyond secure development, implement runtime protection:
Web Application Firewall
Deploy a web application firewall (WAF) to protect against common attacks:
- Block known attack patterns (SQL injection, XSS, etc.)
- Implement rate limiting to prevent abuse
- Create custom rules for application-specific threats
- Regularly update rule sets to address new vulnerabilities
WAFs provide an important additional layer of protection, especially for legacy applications with inherent vulnerabilities.
Security Monitoring
Implement comprehensive security logging and monitoring:
// Basic security logging example
function logSecurityEvent(eventType, userId, resource, success, details) {
logger.info({
type: 'SECURITY',
eventType: eventType,
userId: userId,
resource: resource,
success: success,
details: details,
timestamp: new Date().toISOString()
});
}
- Log security-relevant events (authentication, access control, input validation)
- Implement real-time alerting for critical security events
- Create security dashboards for visibility
- Establish incident response procedures for security events
Effective monitoring enables threat detection and provides forensic information for incident investigation. Organizations should define clear procedures for responding to security incidents discovered through monitoring.
Case Study: Web Security Transformation
A mid-sized financial services company implemented a focused web application security program with remarkable results:
Security Improvements
The company prioritized these key actions:
- Implemented automated security testing in CI/CD pipelines
- Deployed WAF protection for all customer-facing applications
- Created standardized security libraries for development teams
- Established security champions within each development team
Key Outcomes
After six months of implementation:
- Vulnerabilities in new code decreased by 85%
- Security incidents reduced by 70%
- Developer productivity improved through standardized components
- Regulatory compliance status significantly improved
This targeted approach demonstrates how focusing on core security controls can yield significant improvements without massive investment.
Security Program Implementation
Based on our experience, we recommend this approach for implementing web application security:
- Start with an assessment to identify current vulnerabilities and gaps
- Implement foundational controls first (input validation, authentication, TLS)
- Integrate automated testing into development processes
- Deploy runtime protection for production applications
- Develop security knowledge within development teams
- Establish metrics to track security improvement over time
Organizations should prioritize these steps based on their specific risk profile and security maturity. Most organizations should begin with automated security testing and basic security controls to establish a solid foundation.
Conclusion: Building Secure Web Applications
Web application security requires a balanced approach that addresses both development practices and runtime protection. By implementing the strategies and controls outlined in this article, organizations can significantly reduce their vulnerability to web application attacks while supporting business innovation.
The most effective web application security programs focus on a core set of high-impact controls, implement automation to ensure consistent application, and build security knowledge across development teams. This practical approach delivers meaningful security improvements without overwhelming resource constraints.
For more information on securing your web applications, explore our related resources:
- Web Application Security Best Practices
- Top 10 Common Vulnerabilities in Web Applications
- WordPress Security Guide
- Advanced Penetration Testing Techniques
- DevSecOps Integration Guide
Have you implemented web application security controls in your organization? Share your experiences in the comments below or reach out to our team for assistance with securing your web applications.